전체 글
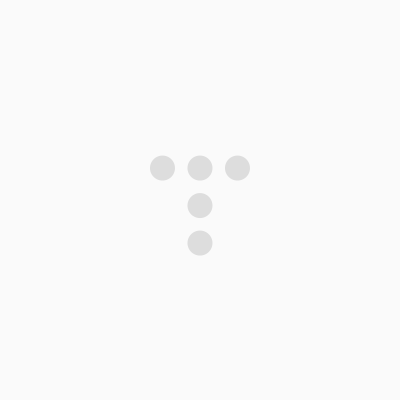
-
import Foundation func solution(_ my_str:String, _ n:Int) -> [String] { let my_str = Array(my_str) var result = [String]() for i in stride(from: 0, to: my_str.count, by: n) { let j = i + n - 1 j < my_str.count ? result.append(my_str[i...j].reduce("") {$0 + String($1)}) : result.append(my_str[i...my_str.count - 1].reduce("") {$0 + String($1)}) } return result }
[Swift] 잘라서 배열로 저장하기import Foundation func solution(_ my_str:String, _ n:Int) -> [String] { let my_str = Array(my_str) var result = [String]() for i in stride(from: 0, to: my_str.count, by: n) { let j = i + n - 1 j < my_str.count ? result.append(my_str[i...j].reduce("") {$0 + String($1)}) : result.append(my_str[i...my_str.count - 1].reduce("") {$0 + String($1)}) } return result }
2023.01.05 -
import Foundation func solution(_ n:Int) -> Int { return Array(String(n)).map { Int(String($0))! }.reduce(0, +) }
[Swift] 자릿수 더하기import Foundation func solution(_ n:Int) -> Int { return Array(String(n)).map { Int(String($0))! }.reduce(0, +) }
2023.01.05 -
func solution(_ myString: String, _ num1: Int, _ num2: Int) -> String { var array = ArraySlice(myString) array.swapAt(num1, num2) return array.map { String($0) }.joined() }
[Swift] 인덱스 바꾸기func solution(_ myString: String, _ num1: Int, _ num2: Int) -> String { var array = ArraySlice(myString) array.swapAt(num1, num2) return array.map { String($0) }.joined() }
2023.01.05 -
import Foundation func solution(_ bin1:String, _ bin2:String) -> String { return String(Int(bin1, radix: 2) + Int(bin2, radix: 2), radix: 2) }
[Swift] 이진수 더하기import Foundation func solution(_ bin1:String, _ bin2:String) -> String { return String(Int(bin1, radix: 2) + Int(bin2, radix: 2), radix: 2) }
2023.01.05 -
import Foundation func gcd(_ a: Int, _ b: Int) -> Int { if b == 0 { return a } else { return gcd (b, a % b) } } func primefactor(_ n:Int) -> Set { var n = n var prime = 2 var result = Set() while n >= prime { if n % prime == 0 { n /= prime result.insert(prime) } else { prime += 1 } } return result } func solution(_ a:Int, _ b:Int) -> Int { let gcdAB = gcd(a,b) let a = a / gcdAB let b = b / gcdAB..
[Swift] 유한소수 판별하기import Foundation func gcd(_ a: Int, _ b: Int) -> Int { if b == 0 { return a } else { return gcd (b, a % b) } } func primefactor(_ n:Int) -> Set { var n = n var prime = 2 var result = Set() while n >= prime { if n % prime == 0 { n /= prime result.insert(prime) } else { prime += 1 } } return result } func solution(_ a:Int, _ b:Int) -> Int { let gcdAB = gcd(a,b) let a = a / gcdAB let b = b / gcdAB..
2023.01.05 -
import Foundation func solution(_ age:Int) -> String { String(age).map { String(UnicodeScalar(97 + Int(String($0))!)!) }.joined() }
[Swift] 외계행성의 나이import Foundation func solution(_ age:Int) -> String { String(age).map { String(UnicodeScalar(97 + Int(String($0))!)!) }.joined() }
2023.01.05 -
import Foundation func solution(_ spell:[String], _ dic:[String]) -> Int { dic.map { String($0.sorted()) }.contains(spell.sorted().joined()) ? 1 : 2 } solution(["s", "o", "m", "d"], ["moos", "dzx", "smm", "sunmmo", "som"])
[Swift] 외계어 사전import Foundation func solution(_ spell:[String], _ dic:[String]) -> Int { dic.map { String($0.sorted()) }.contains(spell.sorted().joined()) ? 1 : 2 } solution(["s", "o", "m", "d"], ["moos", "dzx", "smm", "sunmmo", "som"])
2023.01.05 -
import Foundation func solution(_ babbling:[String]) -> Int { var result = [String]() for babble in babbling { var element = babble element = element.replacingOccurrences(of: "aya", with: "1") element = element.replacingOccurrences(of: "ye", with: "2") element = element.replacingOccurrences(of: "woo", with: "3") element = element.replacingOccurrences(of: "ma", with: "4") result.append(element) }..
[Swift] 옹알이 (1)import Foundation func solution(_ babbling:[String]) -> Int { var result = [String]() for babble in babbling { var element = babble element = element.replacingOccurrences(of: "aya", with: "1") element = element.replacingOccurrences(of: "ye", with: "2") element = element.replacingOccurrences(of: "woo", with: "3") element = element.replacingOccurrences(of: "ma", with: "4") result.append(element) }..
2023.01.05